5.1 KiB
Puppeteer 
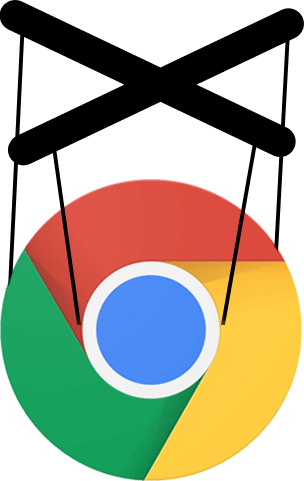
API | FAQ | Contributing
Puppeteer is a node library which provides a high-level API to control headless Chrome over the DevTools Protocol. It can also be configured to use full (non-headless) Chrome.
Use Cases
- Up-to-date testing environment that supports the latest JavaScript features.
- Crawl your site to generate pre-rendered content for your SPA.
- Scrape content from websites.
Installation
To add Puppeteer to your project, run:
yarn add puppeteer
Note
Puppeteer bundles a version of Chromium (~90Mb) which it is guaranteed to work with. However, you're free to point Puppeteer to any Chromium executable (example)
Getting Started
To navigate to https://example.com and save a screenshot as example.png, save the following script as example.js
and run it using node example.js
:
const {Browser} = require('puppeteer');
const browser = new Browser();
browser.newPage().then(async page => {
await page.navigate('https://example.com');
await page.screenshot({path: 'example.png'});
browser.close();
});
A few notes:
- By default, Puppeteer bundles chromium browser with which it works best. However, you can point Puppeteer to a different executable (example)
- Puppeteer creates its own Chromium user profile which it cleans up on every run.
- Puppeteer sets an initial page size to 400px x 300px, which defines the screenshot size. The page size can be changed with
Page.setViewportSize()
method - By default, browser is launched in a headless mode. This could be changed via 'headless' browser option
API Documentation
Explore the API documentation and examples to learn more.
Contributing to Puppeteer
Check out contributing guide to get an overview of puppeteer development.
FAQ
Q: What is Puppeteer?
Puppeteer is a light-weight Node module to control headless Chrome using the DevTools Protocol.
Q: Which Chromium version does Puppeteer use?
Puppeteer bundles chromium it works best with. As chromium improves over time, new versions of puppeteer will be released which depend on a newer chromium versions.
Current chromium version is declared in package.json as chromium_revision
field.
Q: Does Puppeteer work with headless Chromium?
Yes. Puppeteer runs chromium in headless mode by default.
Q: Why do most of the API methods return promises?
Since Puppeteer's code is run by Node, it exists out-of-process to the controlled Chromium instance. This requires most of the API calls to be asynchronous to allow the necessary roundtrips to the browser.
It is recommended to use async/await
to consume asynchronous api:
const {Browser} = require('puppeteer');
const browser = new Browser();
browser.newPage().then(async page => {
await page.setViewport({width: 1000, height: 1000});
await page.pdf({path: 'blank.pdf'});
browser.close();
});
Q: What is the "Phantom Shim"?
To make sure Puppeteer's API is comprehensive, we built PhantomShim - a lightweight phantomJS script runner built atop of Puppeteer API. We run phantomJS tests against PhantomShim with an ultimate goal to pass them all.
To emulate PhantomJS which runs automation scripts in-process to the automated page, PhantomShim spawns nested event loops. On practice, this might result in unpredictable side-effects and makes the shim unreliable, but this works pretty good for testing goals.
Note
It is strictly not recommended to use PhantomShim out in the wild.
Q: What is the difference between Puppeteer and Selenium / WebDriver?
Selenium / WebDriver is a well-established cross-browser API that is useful for testing cross-browser support.
Puppeteer is useful for single-browser testing. For example, many teams only run unit tests with a single browser (e.g. Phantom). In non-testing use cases, Puppeteer provides a powerful but simple API because it's only targeting one browser that enables you to rapidly develop automation scripts.